Detracto erroribus et mea. Malorum temporibus vix ex. Ius ad iudico labores dissentiunt. In eruditi volumus nec nibh blandit deseruisse ne nec, vocibus albucius maluisset ex usu.
Malorum temporibus vix ex.Ius ad iudico labores dissentiunt. In eruditi volumus nec, nibh blandit deseruisse ne nec, vocibus albucius maluisset ex usu.

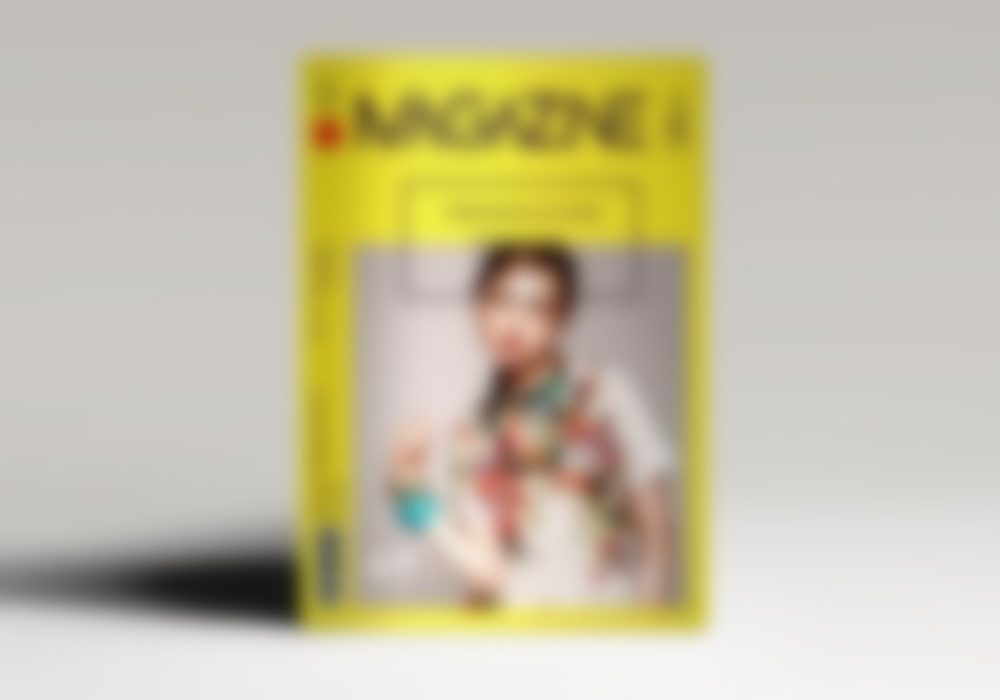
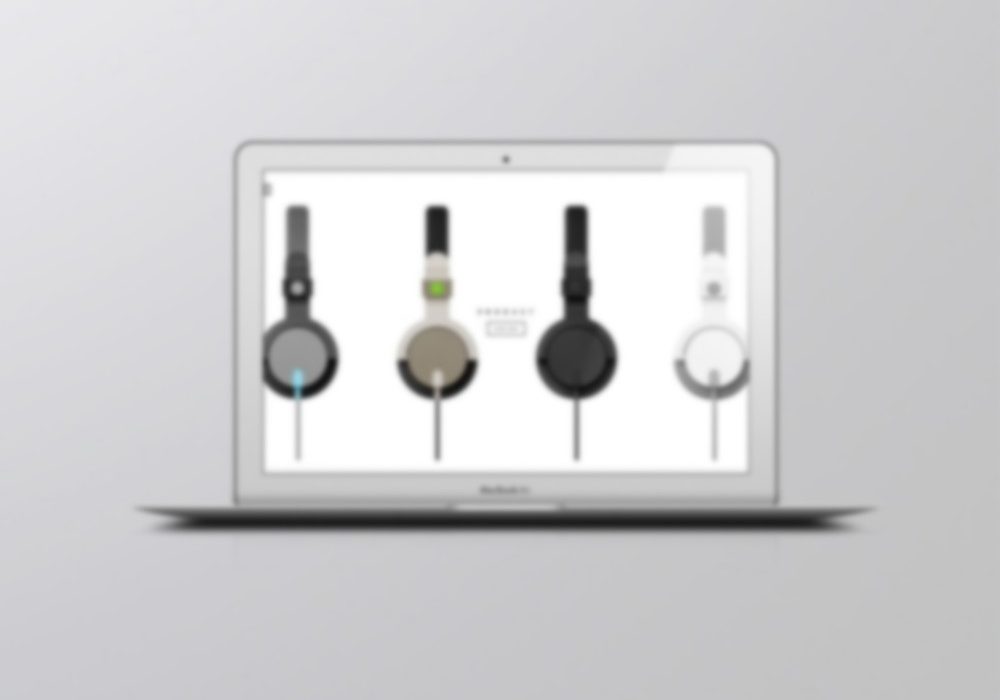
Nihil quaeque moderatius quo ut, eu vix noster fierent postulant. Est ut magna tation, nec timeam tractatos dissentiunt id, ne integre albucius eam. Animal docendi efficiantur ut eam.
Lorem ipsum dolor sit amet, ocurreret assentior sit id, et nam impetus numquam. Detracto erroribus et mea. Malorum temporibus vix ex.
Malorum temporibus vix ex.Ius ad iudico labores dissentiunt. In eruditi volumus nec, nibh blandit deseruisse ne nec, vocibus albucius maluisset ex usu.
Ea mei nostrum imperdiet deterruisset, mei ludus efficiendi ei. Sea summo mazim ex, ea errem eleifend definitionem vim.Malorum temporibus vix ex.
Nihil quaeque moderatius quo ut, eu vix noster fierent postulant. Est ut magna tation, nec timeam tractatos dissentiunt id, ne integre albucius eam. Animal docendi efficiantur ut eam.
Malorum temporibus vix ex. Ius ad iudico labores dissentiunt. In eruditi volumus nec, nibh blandit deseruisse ne nec, vocibus albucius maluisset ex usu.